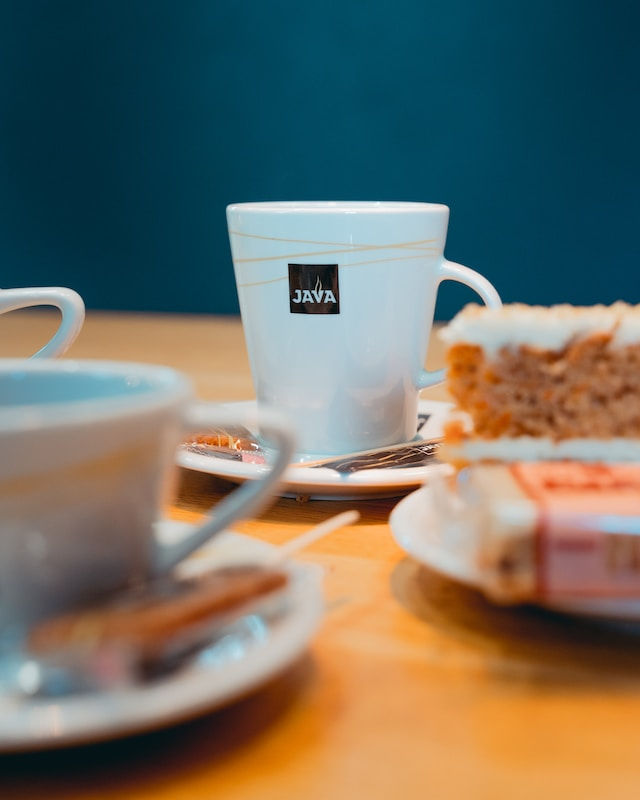
Java, known for its flexibility and robustness, offers native support for data handling through Java records. Project Lombok, on the other hand, presents an alternative approach, aiming to reduce boilerplate code. In this article, we'll explore practical use cases of Java records and Project Lombok, providing comprehensive code examples and comparing their functionalities.
Understanding Java Records:
Java records, introduced in recent versions, simplify the creation of immutable data classes by automating the generation of essential methods.
Understanding Project Lombok:
Project Lombok operates as a library that simplifies Java code by generating repetitive elements like getters, setters, and constructors via annotations.
Let's begin by defining an Employee record using Java records:
public record Employee(String name, int employeeId) { }
The concise Employee record encapsulates employee name and ID effortlessly.
Now, let's achieve the same functionality using Project Lombok:
import lombok.Data;
@Data
public class Employee {
private String name;
private int employeeId;
}
The @Data annotation in Project Lombok generates getters, setters, constructors, and other essential methods for the Employee class.
Use Case 2: Representing Product Information
Next, let's model product details using Java records:
public record Product(String productName, double price) { }
This Java record succinctly defines a Product class with product name and price components.
In comparison, let's employ Project Lombok for a similar Product class:
import lombok.AllArgsConstructor;
import lombok.Getter;
@AllArgsConstructor
@Getter
public class Product {
private String productName;
private double price;
}
Here, annotations like @AllArgsConstructor and @Getter generate constructors and getters for the Product class.
Comparative Analysis:
Java records offer a built-in solution within the Java language, promoting clarity and conciseness without external dependencies. Conversely, Project Lombok relies on annotations and an external library to achieve similar functionalities, potentially introducing an additional dependency.
Conclusion:
Java records and Project Lombok both serve as valuable tools for simplifying data models. While Java records leverage the language's native capabilities, Project Lombok offers a more annotation-driven approach with external support.
Comentarios